Mastering C++ Programming: A Guide to Excelling in Your Assignments
Welcome to ProgrammingHomeworkHelp.com, your ultimate destination for expert assistance with C++ programming assignments. Whether you're a seasoned coder or just dipping your toes into the world of programming, we're here to provide the guidance and support you need to excel in your coursework. In this comprehensive guide, we'll explore key concepts in C++ programming and provide expert solutions to master-level questions, all aimed at helping you tackle your assignments with confidence.
Understanding the Fundamentals of C++ Programming
Before we dive into the master-level questions, let's first establish a solid understanding of the fundamentals of C++ programming. C++ is a powerful and versatile programming language commonly used for developing system software, application software, device drivers, and much more. It combines the features of both high-level and low-level languages, making it an ideal choice for a wide range of applications.
One of the key features of C++ is its support for object-oriented programming (OOP), which allows for the creation of modular and reusable code. Classes and objects are fundamental concepts in C++ OOP, allowing developers to encapsulate data and behavior into cohesive units.
Another important aspect of C++ programming is memory management. Unlike some higher-level languages, C++ gives programmers explicit control over memory allocation and deallocation, which can lead to more efficient and optimized code.
Now that we've covered the basics, let's put our knowledge to the test with some master-level programming questions.
Question 1:
Write a C++ program to implement a binary search tree (BST) and perform the following operations:
Insert a new node into the BST
Delete a node from the BST
Search for a specific value in the BST
Traverse the BST in inorder, preorder, and postorder
Solution:
// C++ program to demonstrate operations of BST
#include <iostream>
using namespace std;
class Node {
public:
int key;
Node* left;
Node* right;
};
Node* newNode(int item) {
Node* temp = new Node();
temp->key = item;
temp->left = temp->right = nullptr;
return temp;
}
Node* insert(Node* root, int key) {
if (root == nullptr) return newNode(key);
if (key < root->key) root->left = insert(root->left, key);
else if (key > root->key) root->right = insert(root->right, key);
return root;
}
// Other functions (deleteNode, search, inorder, preorder, postorder) would be implemented here
int main() {
Node* root = nullptr;
root = insert(root, 50 );
insert(root, 30 );
insert(root, 20 );
insert(root, 40 );
insert(root, 70 );
insert(root, 60 );
insert(root, 80 );
// Other function calls (deleteNode, search, inorder, preorder, postorder) would be made here
return 0;
}
Question 2:
Given an array of integers, write a C++ program to find the maximum subarray sum using the Kadane's algorithm.
Solution:
#include <iostream>
#include <climits>
using namespace std;
int maxSubArraySum(int arr[], int n) {
int max_so_far = INT_MIN, max_ending_here = 0;
for (int i = 0; i < n; i++) {
max_ending_here = max_ending_here + arr[i];
if (max_so_far < max_ending_here)
max_so_far = max_ending_here;
if (max_ending_here < 0 )
max_ending_here = 0;
}
return max_so_far;
}
int main() {
int arr[] = {-2, -3, 4, -1, -2, 1, 5, -3};
int n = sizeof(arr) / sizeof(arr[0]);
int max_sum = maxSubArraySum(arr, n);
cout << "Maximum contiguous sum is " << max_sum;
return 0;
}
Incorporating these solutions into your assignments will not only demonstrate your proficiency in C++ programming but also showcase your ability to tackle complex problems effectively.
At ProgrammingHomeworkHelp.com, we understand that mastering C++ programming can be challenging, which is why we're here to offer expert assistance every step of the way. Whether you need help with C++ programming assignments, projects, or exam preparation, our team of experienced programmers is ready to provide the support you need.
In conclusion, mastering C++ programming requires a solid understanding of its fundamental concepts and the ability to apply them to solve real-world problems. By practicing with master-level questions like the ones provided in this guide and seeking assistance when needed, you can develop the skills and confidence necessary to excel in your C++ programming assignments.
If you're struggling with a C++ programming assignment or simply need some guidance, don't hesitate to reach out to us for help. Our team of experts is standing by to assist you in achieving your academic goals. Remember, at ProgrammingHomeworkHelp.com, we're here to help you succeed. Visit at https://www.programminghomewor....khelp.com/cpp-assign
#programmingassignmenthelp #cppassignmenthelp #helpwithcppprogrammingassignment #college
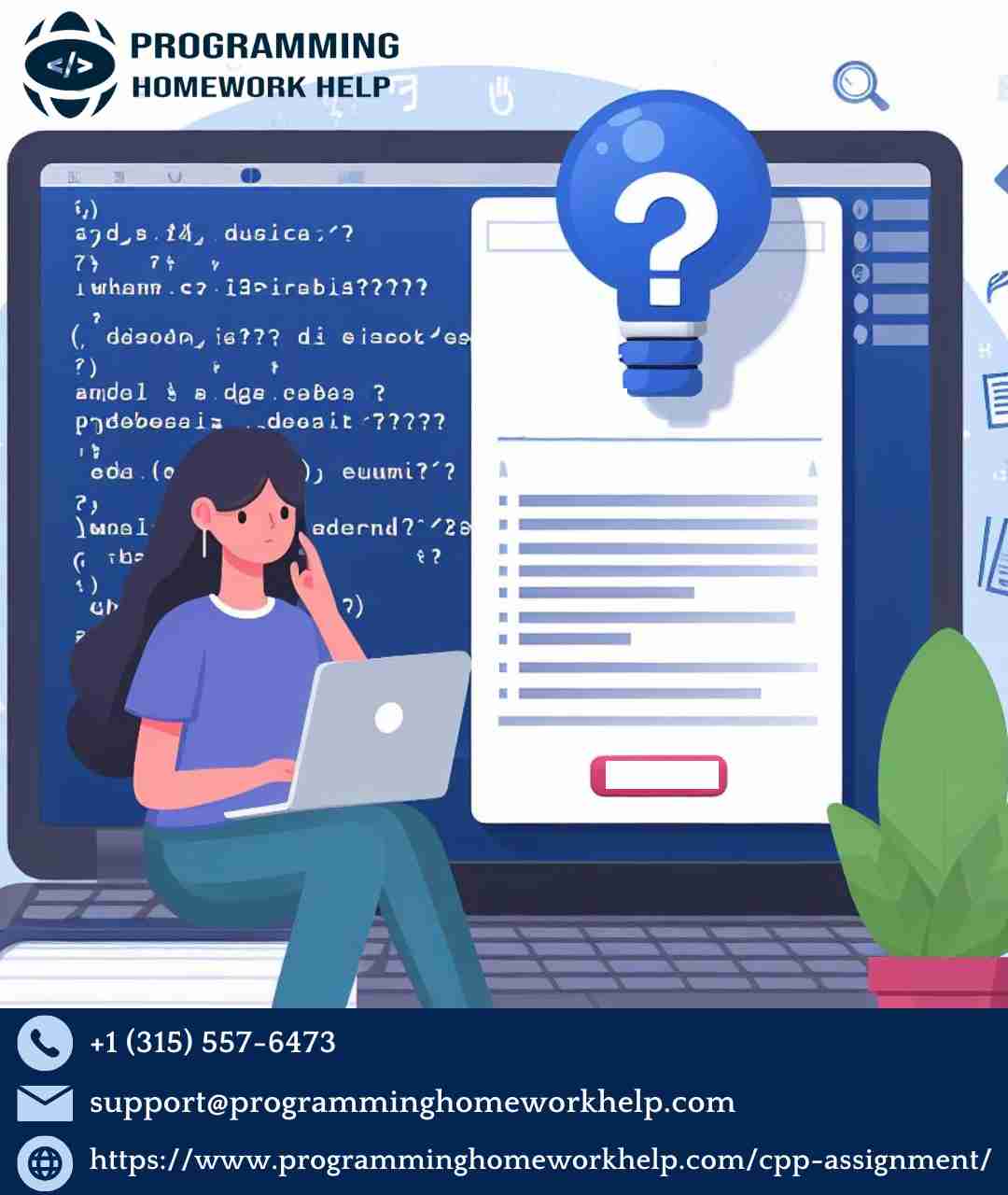